This program allows participants to prepare coursework to develop their Java language skills and prepare for the Oracle Certified Professional, Java SE 8 Programmer Exam.
Aimed at
- Candidates who are interested in continuing their knowledge of Java SE8 and are seeking Java SE 8 Programmer certification.
Duration
40 hours
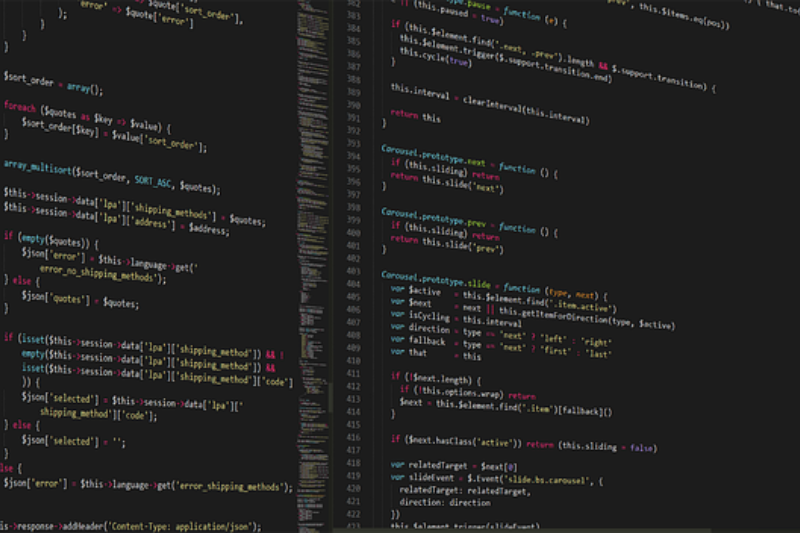
Modalities
- On-site
- Virtual live through digital platforms
- On site
Includes
- 40 hours of training
- Certified instructors
- Participant's Manual
- Proof of participation
Agenda
- Define how the Java language achieves platform independence.
- Differentiate between Java ME, Java SE and Java EE platforms.
- Evaluation of database, middleware and Java library options
- Define how the Java language continues to evolve.
- Create simple Java classes
- Creating primitive variables
- Using operators
- Create and manipulate chains
- Use of if-else and switch statements
- Iterating with loops: while, do-while, for, enhanced for
- Creating arrangements
- Use of Java fields, constructors and methods
- Use of encapsulation in Java class design
- Business problem modeling using Java classes
- Make classes immutable
- Creation and use of Java subclasses.
- Overloading methods
- Use of access levels: private, protected, default and public.
- Primary methods
- Use of virtual method invocation
- Use varargs to specify variable arguments
- Using the instanceof operator to compare object types
- Use upward and downward casts
- Modeling business problems using the static keyword
- Implementation of the singleton design pattern
- Designing general-purpose base classes using abstract classes
- Construction of Java abstract classes and subclasses
- Apply the final keyword in Java
- Distinguishing between higher-level and nested classes
- Definition of a Java interface
- Choosing between interface inheritance and class inheritance
- Extending an interface
- Methods of noncompliance
- Anonymous internal classes
- Definition of a Lambda expression
- Create a custom generic class
- Using the type inference diamond to create an object
- Creating a collection using generics
- ArrayList implementation
- Implementation of a TreeSet
- Implementing a HashMap
- Implementation of a Deque Ordering collections
- Describe the Builder pattern
- Iterating through a collection using lambda syntax
- Stream interface description
- Filtering a collection using lambda expressions
- Calling an existing method using a method reference
- Chaining several methods together
- Definition of pipelines in terms of lambdas and collections.
- List of integrated interfaces included in java.util.function
- Main interfaces: predicate, consumer, function, provider
- Use primitive versions of base interfaces
- Use binary versions of base interfaces
- Extract data from an object using the map
- Describe the types of stream operations
- Describing the optional class
- Describe deferred processing
- Order a sequence
- Saving results in a collection using the collection method
- Grouping and partitioning data using the Collectors class
- Defining the purpose of Java exceptions
- Use of intent and launch statements
- Use of catch clauses, multi-catch clauses and finally
- Automatically close resources with a test statement with resources
- Recognize classes and categories of common exceptions
- Create custom exceptions
- Testing invariants by using assertions
- Create and manage date-based events
- Create and manage time-based events.
- Combine date and time in a single object
- Working with dates and times in different time zones
- Management of changes resulting from daylight saving time
- Definition and creation of timestamps, periods and durations.
- Apply formatting to local and zone dates and times
- Describe the basic concepts of input and output in Java.
- Read and write data from the console
- Use of transmissions to read and write files
- Write and read objects by serialization.
- Using the path interface to operate on file and directory paths
- Use the Files class to check, delete, copy or move a file or directory.
- Using Stream API with NIO2
- Describe the task scheduling of the operating system.
- Create job threads using Runnable and Callable
- Using an ExecutorService to run tasks at the same time
- Identification of possible sub-process problems
- Use of synchronized and concurrent atomics to manage atomicity
- Using monitor locks to control thread execution order
- Using java.util.concurrent.collections
- Parallelism
- The need for Fork-Join
- Labor theft
- RecursiveTask
- Review of the key characteristics of the currents
- Describe how to make a flow pipeline run in parallel.
- List the key assumptions required to use a parallel pipeline.
- Definition of reduction
- Describe why reduction requires an associative function.
- Calculate a value using reduce
- Describe the process for decomposing and then merging the work.
- List key performance considerations for parallel transmissions.
- Describe the basic concepts of input and output in Java.
- JDBC API design definition
- Connection to a database via a JDBC driver
- Send queries and get results from the database.
- Specifying JDBC driver information externally
- Performing CRUD operations using the JDBC API
- Describe the advantages of localizing an application.
- Definition of what a locality represents
- Read and set the locale using the Locale object
- Create a resource package for each location.
- Calling a resource bundle from an application
- Change the regional settings of a resource pack